This article was last updated on December 10, 2024, to add clear introduction and new section to Next.js ENV post..
Introduction
TL;DR: Next.js Environment Variables
In Next.js, environment variables come in very handy for storing API keys/URLs of sensitive nature in a proper and productive manner. They might be set up in files beginning with a dot {.env.} and would be autoloaded in the running Node.js process. You should expose these variables to your browser using the prefix: NEXTPUBLIC.
- Basic Setup: Put variables in .env.local:
API_URL=https://api.example.com
NEXT_PUBLIC_ANALYTICS_ID=UA-123456
Access them in code:
const apiUrl = process.env.API_URL;
const analyticsId = process.env.NEXT_PUBLIC_ANALYTICS_ID
Type: .env.development, .env.production, or .env.test for different environments.
Order of Loading: Next.js checks .env.local first, then .env.
Best Practices: Keep secrets secure, do not commit .env files, and use cloud environment variable settings for production.
Next.js is a React framework that provides many out-of-the-box solutions for building single-page web applications. Under the hood, it handles a lot of tooling and configuration intuitively, making the process of developing applications a breeze.
One of the configurations Next.js handles by default are environment variables. Built-in support for environment variables got a lot easier in Next.js versions 9.4 and later. This means using environment variables in your applications has become seamless and straightforward.
Steps we'll cover:
- What are Environment Variables?
- How to use environment variables in Next.js
- Managing Environment Variables: Best Practices
- Using environment variables in the browser
- Types of Environment Variables in Next.js
- Test Environment Variables
What are Environment Variables?
An environment variable is a key-value pair (key=value) in which a value (or data) is assigned to a key (variable).
Environment variables are used in software development for many use cases; for providing configurations for different application running environments (e.g. development and production environments); for keeping sensitive application data out of application code from malicious users;
The following are valid examples of environment variables:
API_KEY='c4W39R56&gyh_ujij89$'
API_KEY=c4W39R56&gyh_ujij89$
ACCESS_TOKEN='A54EzZoU7o33SByHk1qr'
How to use environment variables in Next.js
Basically, environment variables are added into Next.js apps through a env.local
file. This will then load every variable into the Node.js process. Environment variables in Next.js are only available for use on the server. This means we can only use them in any of Next.js data fetching methods (getServerSideProps, getStaticProps, and getStaticPaths) or API routes.
For example, given a env.local
file in your project root directory with the following content:
API_URL='https://dev.to/api/api/articles/{id}/unpublish'
API_KEY='your_secret_api_key_here'
Next.js will automatically load the variables as process.env.API_URL
and process.env.API_KEY
which we can then use as shown below:
export async function getServerSideProps() {
const response = await axios({
method: "put",
url: process.env.API_URL,
header: {
Authorization: process.env.API_KEY,
},
data: {
id: 12,
},
});
}
NOTE: You should always put the env.local
file in your project root directory to avoid Next.js running into errors.
We can also reference other variables in the same env.local
file using a dollar ($
) sign.
ADMIN_NAME='John'
ADMIN_ID=1234
PROTECTED_URL='https://api.example.com?admin=$ADMIN_NAME&id=$ADMIN_ID'
process.env.PROTECTED_URL
will now evaluate to https://api.example.com?admin=John&id=1234
.
Managing Environment Variables: Best Practices
Managing Environment Variables Securely
Security is crucial when working with environment variables. Here are some best practices:
- Keep Secrets Safe: Use tools like AWS Secrets Manager, Azure Key Vault, or HashiCorp Vault to securely store sensitive information like API keys or database credentials, preventing accidental exposure.
- Encryption: Encrypt
.env
files before sharing. Use a tool likegpg
for encryption:
gpg --symmetric --cipher-algo AES256 .env
- Environment-Specific Variables: Maintain separate files for different environments, such as:
- .env.development for local development.
- .env.production for live apps.
By following these practices, you can minimize the risk of leaks or misuse of sensitive data.
Performance Impact of Environment Variables
Improper use of environment variables can affect your app’s performance. Here’s how to optimize:
- Keep It Light: Only load the variables you need. Avoid adding unused variables to your .env files.
- Static vs. Dynamic Variables: For variables that don’t change at runtime (e.g., API endpoints), load them at build time. For example, in next.config.js:
module.exports = {
env: {
API_URL: "https://api.example.com",
},
};
- Tree-Shaking: Frameworks like Next.js remove unused variables during builds. Clean up unused code to ensure no unnecessary data is included.
Optimizing variable handling improves your app’s performance and reduces overhead.
Cross-Platform Development
Environment variables may behave differently across platforms. Here’s how to maintain consistency:
- Local vs. Cloud: Variables in local .env files won’t automatically work on cloud platforms like Vercel or Netlify. Instead, configure them through the platform’s dashboard.
Example for Vercel: 1. Go to your project settings. 2. Add variables under “Environment Variables.” 3. Deploy your app.
- Docker Support: Use docker-compose.yml to pass variables:
services:
app:
build: .
environment:
- API_URL=https://api.example.com
- Serverless Functions: For serverless environments (e.g., AWS Lambda), configure variables directly in the function settings or use .env files with deployment tools.
By addressing platform-specific differences, you can ensure seamless cross-environment compatibility for your app.
Using environment variables in the browser
Like we mentioned earlier, Next.js env is only available for use on the server by default. However, Next.js provides us a solution to expose the variables to the browser. By adding a NEXT_PUBLIC_
prefix to a variable defined in env.local
Next.js will automatically make it accessible for use in the browser and anywhere else in our application.
For example:
# env.local
NEXT_PUBLIC_GOOGLE_ANALYTICS_ID='your_google_analytics_id_here'
Now we can use it in our code like so:
...
return (
<>
<Script strategy="lazyOnload" src={`https://www.googletagmanager.com/gtag/js?id=${process.env.NEXT_PUBLIC_GOOGLE_ANALYTICS_ID}`} />
<Script strategy="lazyOnload">
{`
window.dataLayer = window.dataLayer || [];
function gtag(){dataLayer.push(arguments);}
gtag('js', new Date());
gtag('config', '${process.env.NEXT_PUBLIC_GOOGLE_ANALYTICS_ID}', {
page_path: window.location.pathname,
});
`}
</Script>
...
</>
)
Here's what is going on above.
In env.local
we defined a NEXT_PUBLIC_GOOGLE_ANALYTICS_ID
variable which holds the value for our Google analytics ID. The prefix NEXT_PUBLIC_
lets us use the variable process.env.NEXT_PUBLIC_GOOGLE_ANALYTICS_ID
in client side code (/pages/_app.js
). Note that _app.js runs on both the client and server.
We added two <Script/>
components from Next.js to add Javascript code to configure google analytics. The <Script/>
component optimizes external scripts that are loaded in a Next.js application by using strategies to improve browser and user experience.
In the previous code, we added lazyOnload
strategy to both scripts; this is to tell the browser we want the analytics to be generated when all other resources have been fetched and the browser is idle.
Types of Environment Variables in Next.js
A single .env.local
file is usually all you need for your environment variables but at times you may want to specify different configurations for different application environments. Next.js allows us to take charge of how we want to configure the variables. There are basically three application environments we'll talk about in this article. These are:
- Development environment (initiated with
next dev
) - Production environment (initiated with
next start
) - Test environment
Default environment variables
A .env
file can be used to specify a default configuration for all three application environments. Note that if the same variables are declared in a .env.local
, it'll override the defaults. .env
and .env.local
files must be added to .gitignore
since they are where sensitive application data are stored.
Development environment variables
You can specify development environment variables in a .env.development
file. This file should be kept in your project directory.
Production environment variables
Use a .env.production
file for environment variables that are particular to production. This file should also be kept in your project directory.
For example, to add environment variables to your live deployments (if you use Vercel to deploy your application), you can add environment variables to a project by going to Settings on your dashboard and clicking on Environment Variables. The UI should look like below:
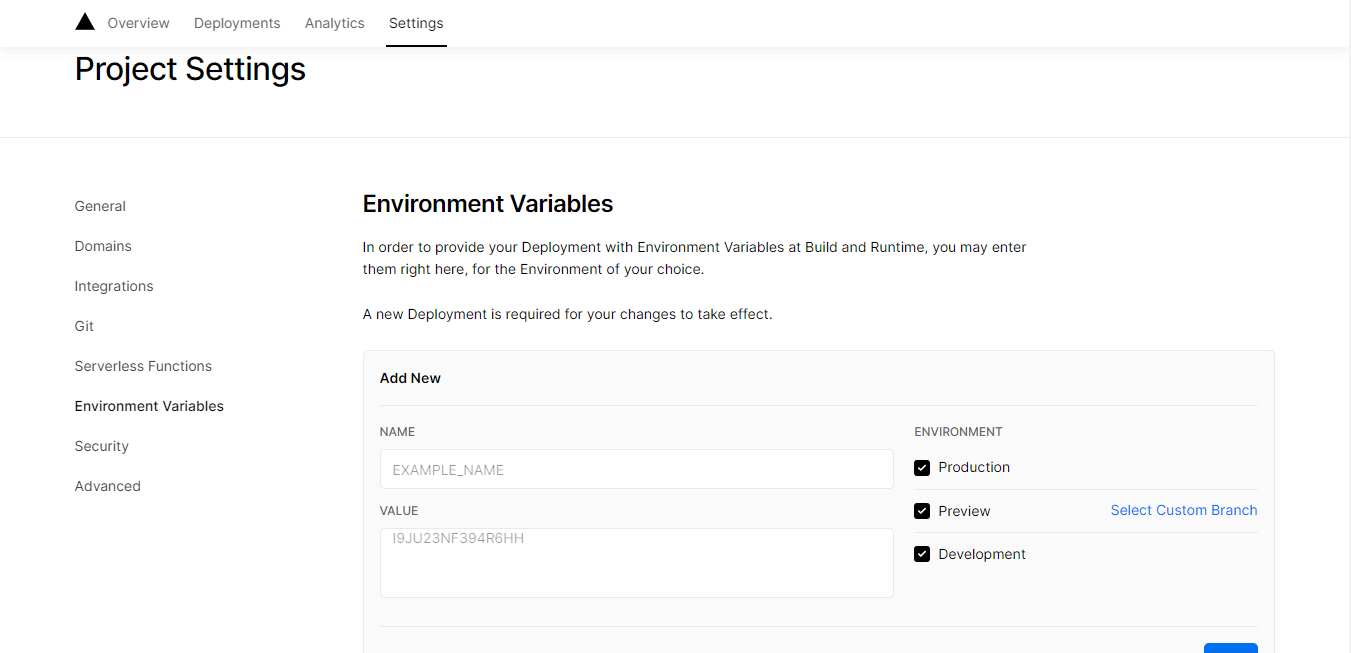
Environment variable load order
Next.js will always search for an environment variable in five places in your application code in the following order until it's found:
process.env
(start).env.$(NODE_ENV).local
(whereNODE_ENV
can be any ofdevelopment
,production
ortest
).env.local
.env.$(NODE_ENV)
(whereNODE_ENV
can be any ofdevelopment
,production
ortest
).env
(end)
So for example if you define a variable in .env.development.local
and then redefine it in env.development
, the value in env.development.local
will be used.
Test Environment Variables
We can also use a .env.test
to specify environment variables for testing purposes. In order to use default test environment variables, you must set NODE_ENV
to test. Here's an example showing how you can do this in package.json
:
...
"scripts": {
"dev": "next dev",
"start": "next start",
"test": "NODE_ENV=test mocha spec"
}
...
Other tools like jest will configure the settings for you by automatically setting the environment to test
.
Note that Next.js will configure your environment variables differently when you're in a test environment. Variables from .env.local
, .env.production
or .env.development
won't be loaded in order for test executions to use the same default configuration. You must include the .env.test
file in your project directory to prevent it from being overridden by .env.local
.
Conclusion
In this article we learned about what environment variables are and how to use them in Next.js. We also implement how to define Next.js env's for different application running environments.