
Auth0 Login
Auth0 is a flexible, drop-in solution for adding authentication and authorization services to your applications. Your team and organization can avoid the cost, time, and risk that comes with building your own solution to authenticate and authorize users. You can check the Auth0 document for details.
We will show you how to use Auth0 with refine
Installation
Run the following command within your project directory to install the Auth0 React SDK:
- npm
- pnpm
- yarn
npm i @auth0/auth0-react
pnpm add @auth0/auth0-react
yarn add @auth0/auth0-react
Configure the Auth0Provider component
Wrap your root component with an Auth0Provider that you can import from the SDK.
import React from "react";
import { createRoot } from "react-dom/client";
import { Auth0Provider } from "@auth0/auth0-react";
import App from "./App";
const container = document.getElementById("root");
const root = createRoot(container!);
root.render(
<React.StrictMode>
<Auth0Provider
domain="YOUR_DOMAIN"
clientId="YOUR_CLIENT_ID"
redirectUri={window.location.origin}
>
<App />
</Auth0Provider>
</React.StrictMode>,
);
Refer to Auth0 docs for detailed configuration.
Override login page
First, we need to override the refine login page. In this way, we will redirect it to the Auth0 login page. We create a login.tsx
file in the /pages
folder.
import { AntdLayout, Button } from "@pankod/refine-antd";
import { useAuth0 } from "@auth0/auth0-react";
export const Login: React.FC = () => {
const { loginWithRedirect } = useAuth0();
return (
<AntdLayout
style={{
background: `radial-gradient(50% 50% at 50% 50%, #63386A 0%, #310438 100%)`,
backgroundSize: "cover",
}}
>
<div style={{ height: "100vh", display: "flex" }}>
<div style={{ maxWidth: "200px", margin: "auto" }}>
<div style={{ marginBottom: "28px" }}>
<img src="./refine.svg" alt="Refine" />
</div>
<Button
type="primary"
size="large"
block
onClick={() => loginWithRedirect()}
>
Sign in
</Button>
</div>
</div>
</AntdLayout>
);
};
After clicking the Login
button, you will be directed to the auth0 login screen.
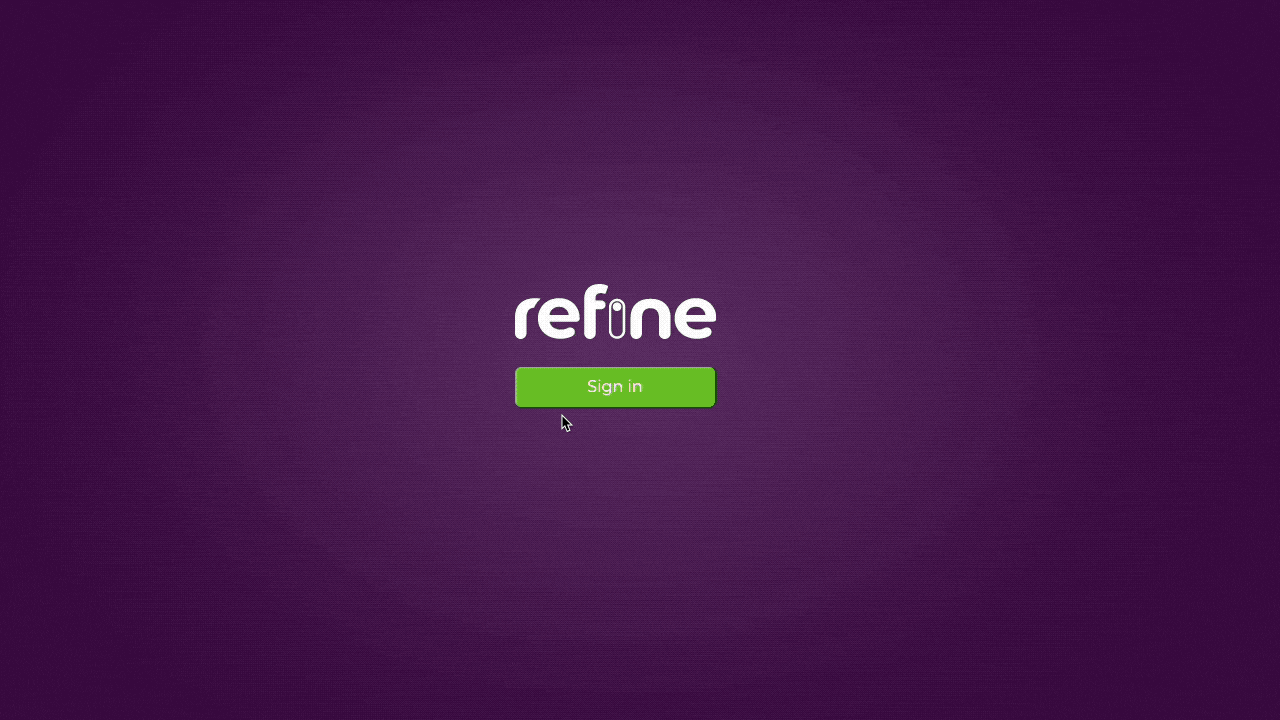
Auth Provider
In refine, authentication and authorization processes are performed with the auth provider. Let's write a provider for Auth0.
import { Refine, AuthProvider } from "@pankod/refine-core";
import {
Layout,
ReadyPage,
useNotificationProvider,
ErrorComponent,
} from "@pankod/refine-antd";
import dataProvider from "@pankod/refine-simple-rest";
import routerProvider from "@pankod/refine-react-router-v6";
import { useAuth0 } from "@auth0/auth0-react";
import "@pankod/refine-antd/dist/reset.css";
import { Login } from "pages/login";
import axios from "axios";
const API_URL = "https://api.fake-rest.refine.dev";
const App = () => {
const { isLoading, isAuthenticated, user, logout, getIdTokenClaims } =
useAuth0();
if (isLoading) {
return <span>loading...</span>;
}
const authProvider: AuthProvider = {
login: () => {
return Promise.resolve();
},
logout: () => {
logout({ returnTo: window.location.origin });
return Promise.resolve("/");
},
checkError: () => Promise.resolve(),
checkAuth: () => {
if (isAuthenticated) {
return Promise.resolve();
}
return Promise.reject();
},
getPermissions: () => Promise.resolve(),
getUserIdentity: () => {
if (user) {
return Promise.resolve({
...user,
avatar: user.picture,
});
}
},
};
getIdTokenClaims().then((token) => {
if (token) {
axios.defaults.headers.common = {
Authorization: `Bearer ${token.__raw}`,
};
}
});
return (
<Refine
LoginPage={Login}
routerProvider={routerProvider}
authProvider={authProvider}
dataProvider={dataProvider(API_URL, axios)}
Layout={Layout}
ReadyPage={ReadyPage}
notificationProvider={useNotificationProvider}
catchAll={<ErrorComponent />}
resources={[
{
name: "posts",
},
]}
/>
);
};
export default App;
Methods
login
loginWithRedirect
method comes from the useAuth0
hook.
logout
logout
method comes from the useAuth0
hook.
checkError & getPermissions
We revert to empty promise because Auth0 does not support it.
checkAuth
We can use the isAuthenticated
method, which returns the authentication status of the useAuth0
hook.
getUserIdentity
We can use it with the user
from the useAuth0
hook.
Example
Auth0 example doesn't work in CodeSandbox embed mode. With this link, you can open the example in the browser and try it.
npm create refine-app@latest -- --example auth-auth0