Custom Inputs
refine uses Ant Design's <Form>
components to control and work with form data. Ant Design supports custom form items inside the <Form.Item>
components. These items should be controllable via their value
property and should implement onChange
(or a custom callback name specified by <Form.Item>
's trigger
prop).
For some data types, displaying and editing as plain text may cause user experience problems.
Custom components may be useful when working with markdown (with markdown editor), JSON based rich text (draft, quill like editors), and HTML (a HTML editor). It can be used in table columns and form fields
Refer to the Ant Design docs for more detailed information about <Form>
. →
Example
We will demonstrate how to use custom input fields for markdown data by adding a markdown editor to edit and create forms.
import React, { useState } from "react";
import { IResourceComponentsProps } from "@pankod/refine-core";
import { Edit, Form, Input, useForm } from "@pankod/refine-antd";
import MDEditor from "@uiw/react-md-editor";
import { IPost } from "interfaces";
export const PostEdit: React.FC = (props) => {
const { formProps, saveButtonProps } = useForm<IPost>();
return (
<Edit {...props} saveButtonProps={saveButtonProps}>
<Form {...formProps} layout="vertical">
<Form.Item
label="Title"
name="title"
rules={[
{
required: true,
},
]}
>
<Input />
</Form.Item>
<Form.Item
label="Content"
name="content"
rules={[
{
required: true,
},
]}
>
<MDEditor data-color-mode="light" />
</Form.Item>
</Form>
</Edit>
);
};
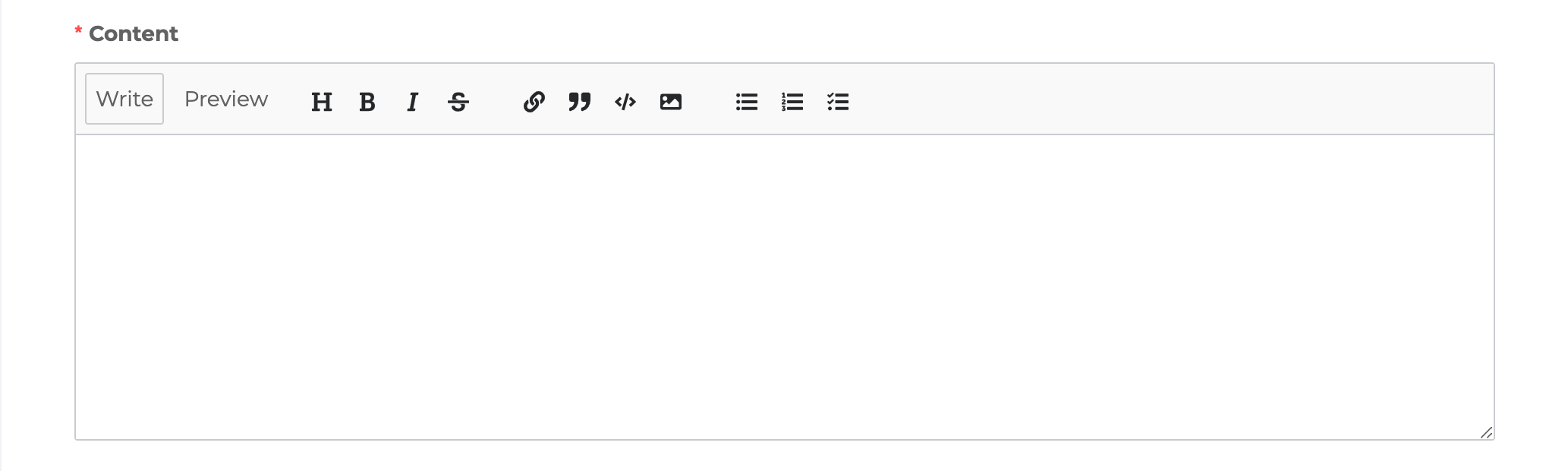
Example
npm create refine-app@latest -- --example input-custom