Strapi-v4
refine supports the features that come with Strapi-v4.
A few of the Strapi-v4 API features are as follows:
- Fields Selection
- Relations Population
- Publication State
- Locale
metaData
allows us to use the above features in hooks. Thus, we can fetch the data according to the parameters we want.
Hooks and components that support metaData
:
NOTE
There is no need to use metaData
for sorting, pagination, and, filters. Sorting, pagination, and, filters will be handled automatically by the strapi-v4 dataProvider.
INFORMATION
Normally, strapi-v4 backend returns data in the following format:
{
"id": 1,
"attributes": {
"title": "My title",
"content": "Long content...",
}
However, we can use normalizeData to customize the data returned by the backend. So, our data will look like:
{
"id": 1,
"title": "My title",
"content": "Long content..."
}
Setup
- npm
- pnpm
- yarn
npm i @pankod/refine-strapi-v4
pnpm add @pankod/refine-strapi-v4
yarn add @pankod/refine-strapi-v4
CAUTION
To make this example more visual, we used the @pankod/refine-antd
package. If you are using Refine headless, you need to provide the components, hooks, or helpers imported from the @pankod/refine-antd
package.
Usage
import { Refine, AuthProvider } from "@pankod/refine-core";
import routerProvider from "@pankod/refine-react-router-v6";
import { DataProvider } from "@pankod/refine-strapi-v4";
import routerProvider from "@pankod/refine-react-router-v6";
const App: React.FC = () => {
return (
<Refine
authProvider={authProvider}
dataProvider={DataProvider("API_URL")}
routerProvider={routerProvider}
Layout={Layout}
ReadyPage={ReadyPage}
notificationProvider={useNotificationProvider}
catchAll={<ErrorComponent />}
/>
);
};
API Parameters
Let's examine how API parameters that come with Strapi-v4 are used with metaData
. Then, let's see how it is used in the application.
Create Collections
We created two collections on Strapi as posts
and categories
and added a relation between them. For detailed information on how to create a collection, you can check here.
- posts
- categories
posts
posts has the following fields:
id
title
content
category
createdAt
locale
categories
categories has the following fields:
id
title
Fields Selection
To select only some fields, we must specify these fields with `metaData``.
Refer to the Fields Selection documentation for detailed information. →
const { tableProps } = useTable<IPost>({
metaData: {
fields: ["id", "title"],
},
});
const { tableProps } = useTable<IPost>({
metaData: {
fields: "*",
},
});
When sending the request, we can specify which fields will come, so we send fields
in metaData
to hooks that we will fetch data from. In this way, you can perform the queries of only the fields you want.
import { useState } from "react";
import { IResourceComponentsProps } from "@pankod/core";
import {
List,
Table,
useTable,
getDefaultSortOrder,
FilterDropdown,
Select,
useSelect,
Space,
EditButton,
DeleteButton,
} from "@pankod/refine-antd";
import { IPost } from "interfaces";
import { API_URL } from "../../constants";
export const PostList: React.FC<IResourceComponentsProps> = () => {
const { tableProps, sorter } = useTable<IPost>({
metaData: {
fields: ["id", "title"],
},
});
return (
<List>
<Table
{...tableProps}
rowKey="id"
pagination={{
...tableProps.pagination,
showSizeChanger: true,
}}
>
<Table.Column
dataIndex="id"
title="ID"
defaultSortOrder={getDefaultSortOrder("id", sorter)}
sorter={{ multiple: 3 }}
/>
<Table.Column
dataIndex="title"
title="Title"
defaultSortOrder={getDefaultSortOrder("title", sorter)}
sorter={{ multiple: 2 }}
/>
<Table.Column<{ id: string }>
title="Actions"
render={(_, record) => (
<Space>
<EditButton hideText size="small" recordItemId={record.id} />
<DeleteButton hideText size="small" recordItemId={record.id} />
</Space>
)}
/>
</Table>
</List>
);
};
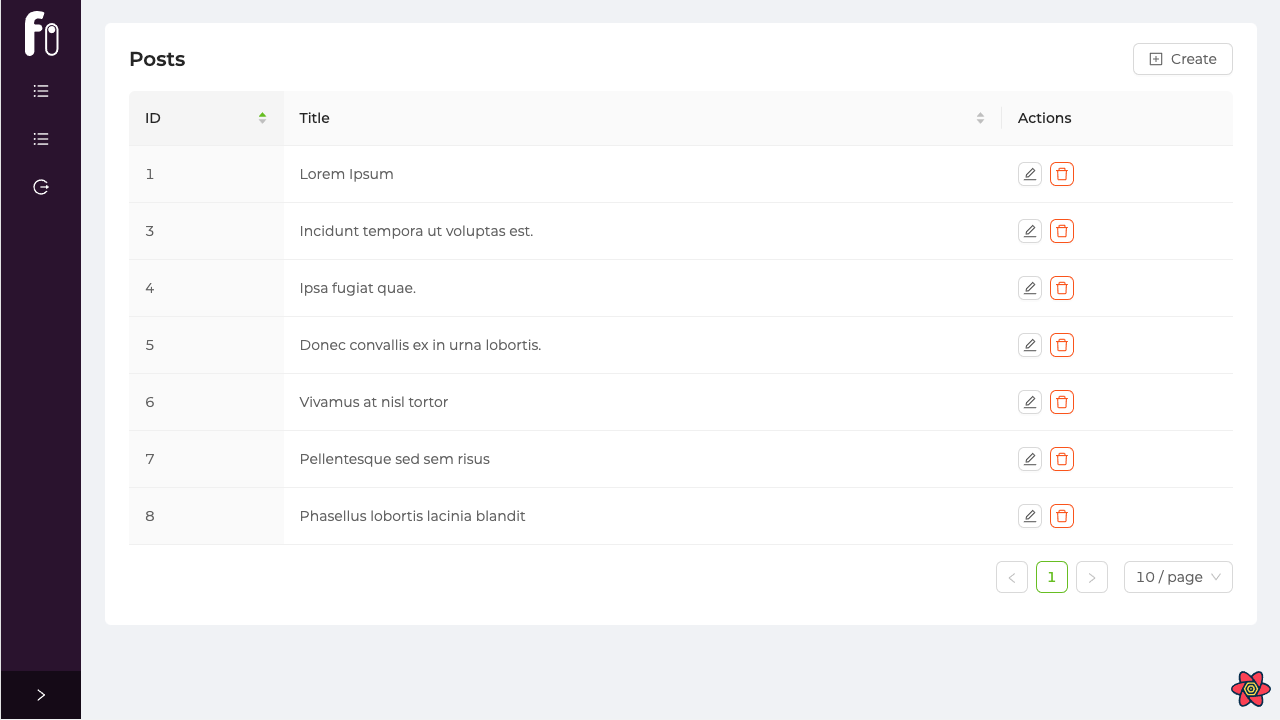
Relations Population
By default, relations are not populated when fetching entries.
The populate
parameter is used to define which fields will be populated.
Refer to the Relations Population documentation for detailed information. →
const { tableProps } = useTable<IPost>({
metaData: {
populate: ["category", "cover"],
},
});
const { tableProps } = useTable<IPost>({
metaData: {
populate: "*",
},
});
It should be noted that Strapi-V4 allows populating relations more than 1 level.
const { tableProps } = useTable<IPost>({
metaData: {
populate: {
category: {
populate: ["cover"],
},
cover: {
populate: [""],
},
},
},
});
In order to pull the categories
related to the posts, we can now show the categories in our list by defining the metaData
populate
parameter.
import { IResourceComponentsProps } from "@pankod/refine-core";
import {
List,
Table,
useTable,
getDefaultSortOrder,
FilterDropdown,
Select,
useSelect,
Space,
EditButton,
DeleteButton,
} from "@pankod/refine-antd";
import { IPost } from "interfaces";
import { API_URL } from "../../constants";
export const PostList: React.FC<IResourceComponentsProps> = () => {
const { tableProps, sorter } = useTable<IPost>({
metaData: {
fields: ["id", "title"],
populate: ["category"],
},
});
const { selectProps } = useSelect({
resource: "categories",
optionLabel: "title",
optionValue: "id",
});
return (
<List>
<Table
{...tableProps}
rowKey="id"
pagination={{
...tableProps.pagination,
showSizeChanger: true,
}}
>
<Table.Column
dataIndex="id"
title="ID"
defaultSortOrder={getDefaultSortOrder("id", sorter)}
sorter={{ multiple: 3 }}
/>
<Table.Column
dataIndex="title"
title="Title"
defaultSortOrder={getDefaultSortOrder("title", sorter)}
sorter={{ multiple: 2 }}
/>
<Table.Column
dataIndex={["category", "title"]}
title="Category"
filterDropdown={(props) => (
<FilterDropdown {...props}>
<Select
style={{ minWidth: 200 }}
mode="multiple"
placeholder="Select Category"
{...selectProps}
/>
</FilterDropdown>
)}
/>
<Table.Column<{ id: string }>
title="Actions"
render={(_, record) => (
<Space>
<EditButton hideText size="small" recordItemId={record.id} />
<DeleteButton hideText size="small" recordItemId={record.id} />
</Space>
)}
/>
</Table>
</List>
);
};
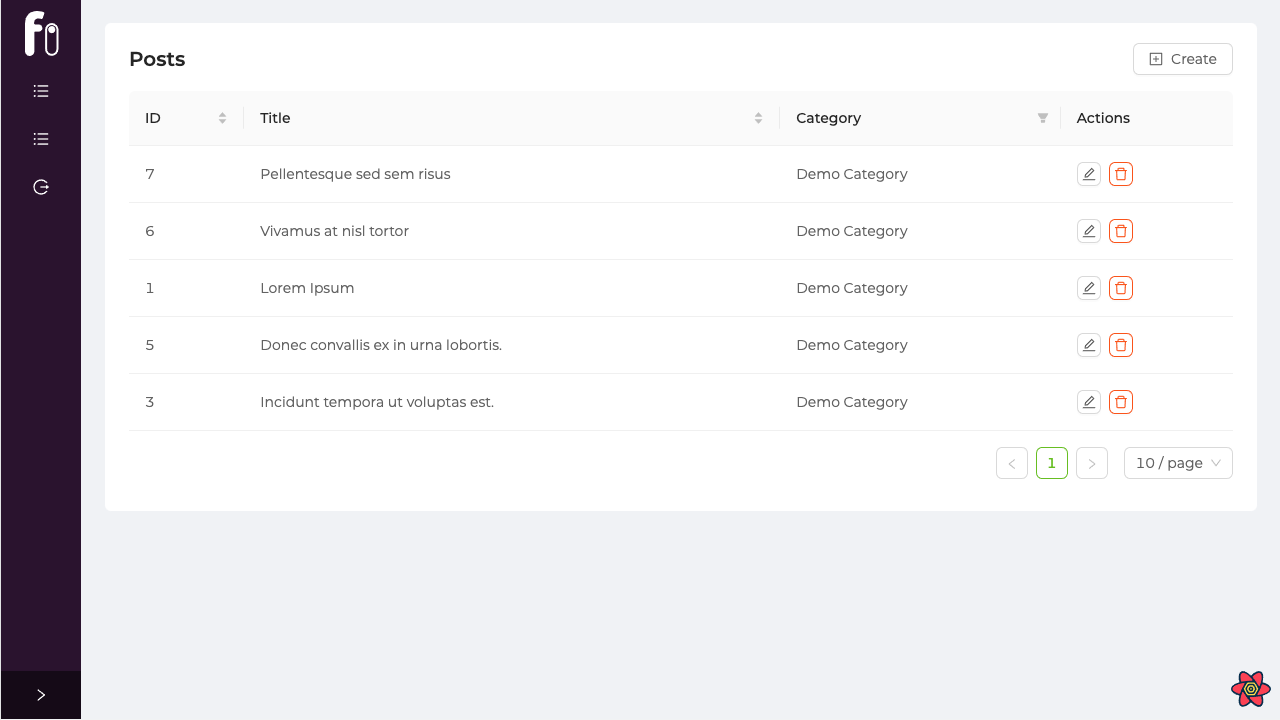
Relations Population for /me
request
If you need to the population for the /me
request you can use it like this in your authProvider
.
const strapiAuthHelper = AuthHelper(API_URL + "/api");
strapiAuthHelper.me("token", {
metaData: {
populate: ["role"],
},
});
Publication State
NOTE
The Draft & Publish feature should be enabled on Strapi.
Refer to the Publication State documentation for detailed information. →
live
: returns only published entries
preview
: returns draft and published entries
const { tableProps } = useTable<IPost>({
metaData: {
publicationState: "preview",
},
});
We can list the posts separately according to the published
or draft
information.
import { useState } from "react";
import { IResourceComponentsProps } from "@pankod/refine-core";
import {
List,
Table,
useTable,
getDefaultSortOrder,
FilterDropdown,
Select,
useSelect,
DateField,
Space,
EditButton,
DeleteButton,
Form,
Radio,
Tag,
} from "@pankod/refine-antd";
import { IPost } from "interfaces";
import { API_URL } from "../../constants";
export const PostList: React.FC<IResourceComponentsProps> = () => {
const [publicationState, setPublicationState] = useState("live");
const { tableProps, sorter } = useTable<IPost>({
metaData: {
fields: ["id", "title"],
populate: ["category"],
publicationState,
},
});
const { selectProps } = useSelect({
resource: "categories",
optionLabel: "title",
optionValue: "id",
});
return (
<List>
<Form
layout="inline"
initialValues={{
publicationState,
}}
>
<Form.Item label="Publication State" name="publicationState">
<Radio.Group onChange={(e) => setPublicationState(e.target.value)}>
<Radio.Button value="live">Published</Radio.Button>
<Radio.Button value="preview">Draft and Published</Radio.Button>
</Radio.Group>
</Form.Item>
</Form>
<br />
<Table
{...tableProps}
rowKey="id"
pagination={{
...tableProps.pagination,
showSizeChanger: true,
}}
>
<Table.Column
dataIndex="id"
title="ID"
defaultSortOrder={getDefaultSortOrder("id", sorter)}
sorter={{ multiple: 3 }}
/>
<Table.Column
dataIndex="title"
title="Title"
defaultSortOrder={getDefaultSortOrder("title", sorter)}
sorter={{ multiple: 2 }}
/>
<Table.Column
dataIndex={["category", "title"]}
title="Category"
filterDropdown={(props) => (
<FilterDropdown {...props}>
<Select
style={{ minWidth: 200 }}
mode="multiple"
placeholder="Select Category"
{...selectProps}
/>
</FilterDropdown>
)}
/>
<Table.Column
dataIndex="publishedAt"
title="Status"
render={(value) => {
return (
<Tag color={value ? "green" : "blue"}>
{value ? "Published" : "Draft"}
</Tag>
);
}}
/>
<Table.Column<{ id: string }>
title="Actions"
render={(_, record) => (
<Space>
<EditButton hideText size="small" recordItemId={record.id} />
<DeleteButton hideText size="small" recordItemId={record.id} />
</Space>
)}
/>
</Table>
</List>
);
};
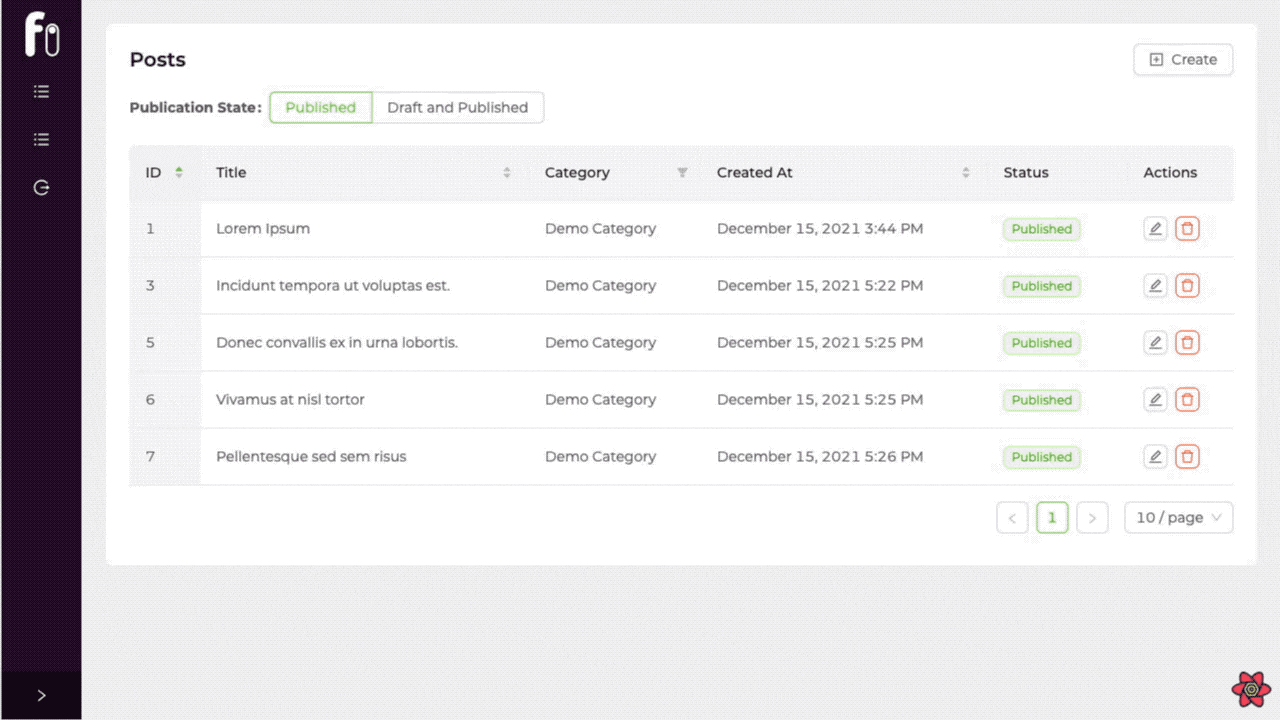
Locale
TIP
To fetch content for a locale, make sure it has been already added to Strapi in the admin panel
Refer to the Locale documentation for detailed information. →
const { tableProps } = useTable<IPost>({
metaData: {
locale: "de",
},
});
With the local parameter feature, we can fetch posts and categories created according to different languages.
import { useState } from "react";
import { IResourceComponentsProps } from "@pankod/refine-core";
import {
List,
Table,
useTable,
getDefaultSortOrder,
FilterDropdown,
Select,
useSelect,
Space,
EditButton,
DeleteButton,
Form,
Radio,
Tag,
} from "@pankod/refine-antd";
import { IPost } from "interfaces";
import { API_URL } from "../../constants";
export const PostList: React.FC<IResourceComponentsProps> = () => {
const [locale, setLocale] = useState("en");
const [publicationState, setPublicationState] = useState("live");
const { tableProps, sorter } = useTable<IPost>({
metaData: {
populate: ["category", "cover"],
locale,
publicationState,
},
});
const { selectProps } = useSelect({
resource: "categories",
optionLabel: "title",
optionValue: "id",
metaData: { locale },
});
return (
<List>
<Form
layout="inline"
initialValues={{
locale,
publicationState,
}}
>
<Form.Item label="Locale" name="locale">
<Radio.Group onChange={(e) => setLocale(e.target.value)}>
<Radio.Button value="en">English</Radio.Button>
<Radio.Button value="de">Deutsch</Radio.Button>
</Radio.Group>
</Form.Item>
<Form.Item label="Publication State" name="publicationState">
<Radio.Group onChange={(e) => setPublicationState(e.target.value)}>
<Radio.Button value="live">Published</Radio.Button>
<Radio.Button value="preview">Draft and Published</Radio.Button>
</Radio.Group>
</Form.Item>
</Form>
<br />
<Table
{...tableProps}
rowKey="id"
pagination={{
...tableProps.pagination,
showSizeChanger: true,
}}
>
<Table.Column
dataIndex="id"
title="ID"
defaultSortOrder={getDefaultSortOrder("id", sorter)}
sorter={{ multiple: 3 }}
/>
<Table.Column
dataIndex="title"
title="Title"
defaultSortOrder={getDefaultSortOrder("title", sorter)}
sorter={{ multiple: 2 }}
/>
<Table.Column
dataIndex={["category", "title"]}
title="Category"
filterDropdown={(props) => (
<FilterDropdown {...props}>
<Select
style={{ minWidth: 200 }}
mode="multiple"
placeholder="Select Category"
{...selectProps}
/>
</FilterDropdown>
)}
/>
<Table.Column
dataIndex="publishedAt"
title="Status"
render={(value) => {
return (
<Tag color={value ? "green" : "blue"}>
{value ? "Published" : "Draft"}
</Tag>
);
}}
/>
<Table.Column<{ id: string }>
title="Actions"
render={(_, record) => (
<Space>
<EditButton hideText size="small" recordItemId={record.id} />
<DeleteButton hideText size="small" recordItemId={record.id} />
</Space>
)}
/>
</Table>
</List>
);
};
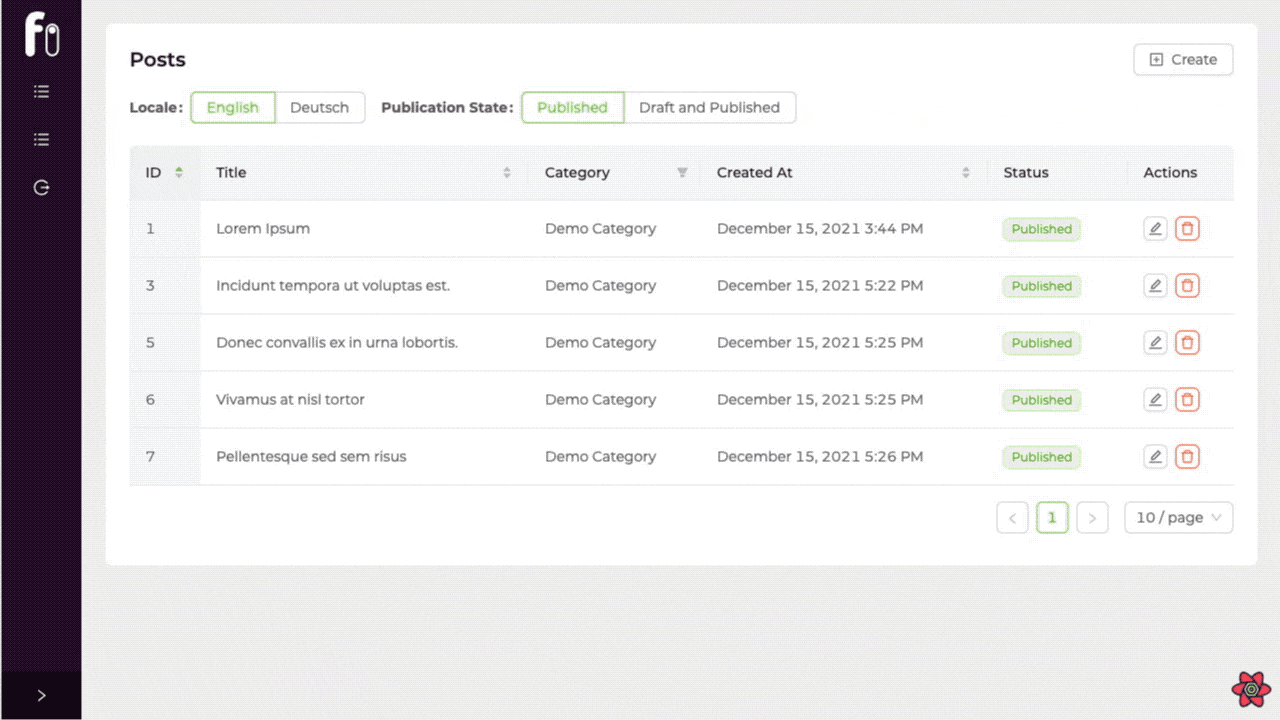
metaData
Usages
When creating and editing posts you can use these API parameters in metaData
:
const { formProps, saveButtonProps, queryResult } = useForm<IPost>({
metaData: { publicationState: "preview" },
});
const { formProps, saveButtonProps, queryResult } = useForm<IPost>({
metaData: { populate: ["category", "cover"] },
});
const { selectProps } = useSelect({
metaData: { locale: "en" },
});
Example
Demo Credentials
Username: demo@refine.dev
Password: demodemo
npm create refine-app@latest -- --example data-provider-strapi-v4