1. Data Provider
The data provider unit is optional for the tutorial and can be skipped to next unit - Adding CRUD Pages if desired.
What is data provider?
The data provider acts as a data layer for your app that makes the HTTP requests and encapsulates how the data is retrieved. refine consumes these methods via data hooks.
You don't need worry about creating data providers from scratch. refine offers built-in data provider support for the most popular API providers. So you can use one of them or you can create your own data provider according to your needs.
We'll see how to create data provider in the next sections.
Data providers can communicate with REST
, GraphQL
, RPC
, and SOAP
based API's. You can imagine the data provider is an adapter between refine and the API.
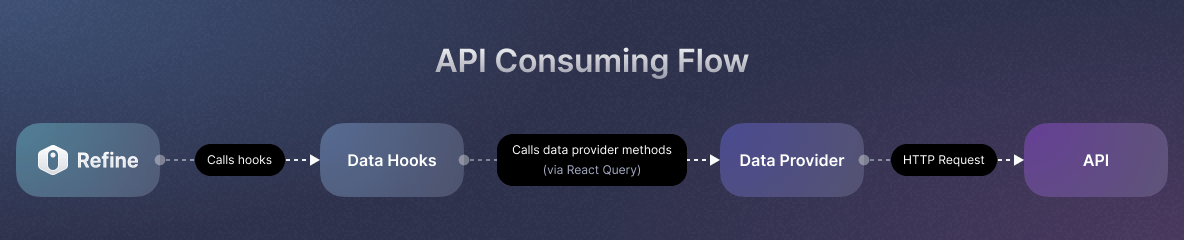
The typical data provider has following methods:
import { DataProvider } from "@pankod/refine-core";
const dataProvider: DataProvider = {
create: ({ resource, variables, metaData }) => Promise,
deleteOne: ({ resource, id, variables, metaData }) => Promise,
getList: ({
resource,
pagination,
hasPagination,
sort,
filters,
metaData,
}) => Promise,
getOne: ({ resource, id, metaData }) => Promise,
update: ({ resource, id, variables, metaData }) => Promise,
getApiUrl: () => "",
...
}
These methods are used to perform data operations by refine.
refine comes with different data providers out of the box, but the one we’re interested in and will be using in this tutorial is the refine-simple-rest
data provider, a data provider for communicating with RESTful APIs.
Using Data Providers in refine
In the previous units, we consumed the API and show the data in the auto-generated Inferencer pages. To allow refine to communicate with the API, we registered a data provider using dataProvider
property of the <Refine>
component.
...
import dataProvider from "@pankod/refine-simple-rest";
<Refine
...
dataProvider={dataProvider("https://api.fake-rest.refine.dev")}
/>;
You can refer to the refine component dataProvider prop documentation for more detailed information.
How are data provider methods used in the app?
We use refine's data hooks whenever we need to fetch data from the API. These data hooks are connected to data provider methods internally. The required parameters are passed to the data provider methods, and the response from the API is returned.
To illustrate this internal connection, imagine we want to get all records from the post
resource using refine's useList
data hook.
import { useList } from "@pankod/refine-core";
const postUseListResult = useList({
resource: "posts",
config: {
sort: [
{
field: "id",
order: "desc",
},
],
filters: [
{
field: "title",
operator: "contains",
value: "hello",
},
],
},
});
As we can see, the parameters passed to the useList
hook are forwarded to the data provider's getList
method internally. In the background, refine connects all data provider methods to appropriate data hooks.
const dataProvider = {
getList: (params) => {
console.log(params);
/*
{
"resource": "posts",
"sort": [
{
"field": "id",
"order": "desc"
}
],
"filters": [
{
"field": "title",
"operator": "contains",
"value": "hello"
}
],
}
*/
}
...
}
Supported Data Providers
Refine supports many data providers. To include them in your project, you can use npm install [packageName]
or you can select the preferred data provider with the npm create refine-app@latest projectName
during the project creation phase with CLI. This will allow you to easily use these data providers in your project.
Community ❤️
- Firebase by rturan29
- Directus by tspvivek
- Elide by chirdeeptomar
- Elide GraphQL by chirdeeptomar
- useGenerated by usegen
- Hygraph by acomagu
- Sanity by hirenf14
- SQLite by mateusabelli
- JSON:API by mahirmahdi
- PocketBase by kruschid
If you have created a custom data provider and would like to share it with the community, please don't hesitate to get in touch with us. We would be happy to include it on this page for others to use.