
<AutoSaveIndicator />
Refine's forms provide a built-in auto-save feature. This allows you to automatically save the form when the user makes changes to the form which can be useful for forms that are long or complex and the user may not want to lose their progress.
The <AutoSaveIndicator />
component is a utility component that can be used to show a visual indicator to the user about the auto-save status of the form.
Refine's core
useForm
hook does not automatically trigger the auto-save feature. You need to manually trigger theonFinishAutoSave
function returned from theuseForm
hook to trigger the auto-save feature.Extended implementations of Refine's
useForm
such as;@refinedev/antd
'suseForm
,@refinedev/react-hook-form
'suseForm
and@refinedev/mantine
'suseForm
automatically trigger the auto-save feature when a form value changes.The
<AutoSaveIndicator />
component is only designed to display a visual feedback to the user about the auto-save status of the form. It does not contain any logic to trigger the auto-save feature.To learn more about the auto-save feature check out Auto Save section in Forms guide
Usage
Usage is as simple as spreading the autoSaveProps
object returned from the useForm
hook into the <AutoSaveIndicator />
component. It will automatically determine the auto-save status and display the appropriate indicator.
import { AutoSaveIndicator, useForm } from "@refinedev/core";
const EditPage = () => {
const { autoSaveProps } = useForm({
autoSave: {
enabled: true,
},
});
console.log(autoSaveProps);
/*
{
status: "success", // "loading" | "error" | "idle" | "success"
error: null, // HttpError | null
data: { ... }, // UpdateResponse | undefined,
}
*/
return (
<div>
{/* We'll pass the autoSaveProps from useForm's response to the <AutoSaveIndicator /> component. */}
<AutoSaveIndicator {...autoSaveProps} />
<form
// ...
>
{/* ... */}
</form>
</div>
);
};
Example below shows the <AutoSaveIndicator />
component in action.
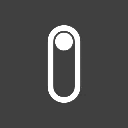
Dependencies: @refinedev/core@latest,@refinedev/simple-rest@latest,@refinedev/react-router@latest,react-router@^7.0.2
Code Files
Customizing the indicator
The <AutoSaveIndicator />
component accepts an elements
prop which can be used to customize the indicator for each status.
import { AutoSaveIndicator, useForm } from "@refinedev/core";
const EditPage = () => {
const { autoSaveProps } = useForm({
autoSave: {
enabled: true,
},
});
return (
<div>
<AutoSaveIndicator
{...autoSaveProps}
elements={{
loading: <span>saving...</span>,
error: <span>auto save error.</span>,
idle: <span>waiting for changes.</span>,
success: <span>saved.</span>,
}}
/>
{/* ... */}
</div>
);
};
API Reference
Properties
Property | Type | Description |
---|---|---|
data |
| The data returned by the update request. |
error |
| The error returned by the update request. |
status ﹡ |
| The status of the update request. |
elements |
| The elements to display for each status. |