
Introduction
Refine provides an integration package for Chakra UI framework. This package provides a set of ready to use components and hooks that connects Refine with Chakra UI components. While Refine's integration offers a set of components and hooks, it is not a replacement for the Chakra UI package, you will be able to use all the features of Chakra UI in the same way you would use it in a regular React application. Refine's integration only provides components and hooks for an easier usage of Chakra UI components in combination with Refine's features and functionalities.
Dependencies: @refinedev/chakra-ui@^2.26.17,@tabler/icons-react@^3.1.0,@refinedev/core@^4.45.1,@refinedev/react-router@latest,@refinedev/simple-rest@^4.5.4,@refinedev/react-table@^5.6.4,@tanstack/react-table@^8.2.6,@refinedev/react-hook-form@^4.8.12,@chakra-ui/react@^2.5.1,react-dom@^18.0.0,react-router@^7.0.2,react-hook-form@^7.43.5
Code Files
Installation
Installing the package is as simple as just by running the following command without any additional configuration:
- npm
- pnpm
- yarn
npm i @refinedev/chakra-ui @chakra-ui/react @refinedev/react-table @refinedev/react-hook-form @tanstack/react-table react-hook-form @tabler/icons-react
pnpm add @refinedev/chakra-ui @chakra-ui/react @refinedev/react-table @refinedev/react-hook-form @tanstack/react-table react-hook-form @tabler/icons-react
yarn add @refinedev/chakra-ui @chakra-ui/react @refinedev/react-table @refinedev/react-hook-form @tanstack/react-table react-hook-form @tabler/icons-react
Usage
We'll wrap our app with the <ChakraProvider />
to make sure we have the theme available for our app, then we'll use the layout components to wrap them around our routes. Check out the examples below to see how to use Refine's Chakra UI integration.
- React Router
- Next.js
- Remix
Dependencies: @refinedev/chakra-ui@^2.26.17,@tabler/icons-react@^3.1.0,@refinedev/core@^4.45.1,@refinedev/react-router@latest,@refinedev/simple-rest@^4.5.4,@refinedev/react-table@^5.6.4,@tanstack/react-table@^8.2.6,@refinedev/react-hook-form@^4.8.12,@chakra-ui/react@^2.5.1,react-dom@^18.0.0,react-router@^7.0.2
Code Files
Dependencies: @refinedev/chakra-ui@^2.26.17,@tabler/icons-react@^3.1.0,@refinedev/core@^4.45.1,@refinedev/react-router@latest,@refinedev/simple-rest@^4.5.4,@refinedev/react-table@^5.6.4,@tanstack/react-table@^8.2.6,@refinedev/react-hook-form@^4.8.12,@chakra-ui/react@^2.5.1,@refinedev/nextjs-router@latest
Code Files
Dependencies: @refinedev/chakra-ui@^2.26.17,@tabler/icons-react@^3.1.0,@refinedev/core@^4.45.1,@refinedev/react-router@latest,@refinedev/simple-rest@^4.5.4,@refinedev/react-table@^5.6.4,@tanstack/react-table@^8.2.6,@refinedev/react-hook-form@^4.8.12,@chakra-ui/react@^2.5.1,@refinedev/remix-router@latest
Code Files
Tables
Chakra UI offers styled table primitives but lacks the table management solution. Refine recommends using @refinedev/react-table
package which is built on top of Refine's useTable
hook and Tanstack Table's useTable
hook to enable features from pagination to sorting and filtering. Refine's documentations and examples of Chakra UI uses @refinedev/react-table
package for table management but you have the option to use any table management solution you want.
import React from "react";
import { useTable } from "@refinedev/react-table";
import { ColumnDef, flexRender } from "@tanstack/react-table";
import { GetManyResponse, useMany } from "@refinedev/core";
import {
List,
ShowButton,
EditButton,
DeleteButton,
DateField,
} from "@refinedev/chakra-ui";
import {
Table,
Thead,
Tbody,
Tr,
Th,
Td,
TableContainer,
HStack,
Text,
} from "@chakra-ui/react";
import { Pagination } from "../../components/pagination";
const columns = [
{ id: "id", header: "ID", accessorKey: "id" },
{
id: "name",
header: "Name",
accessorKey: "name",
meta: { filterOperator: "contains" },
},
{ id: "price", header: "Price", accessorKey: "price" },
{
id: "actions",
header: "Actions",
accessorKey: "id",
enableColumnFilter: false,
enableSorting: false,
cell: function render({ getValue }) {
return (
<HStack>
<ShowButton hideText size="sm" recordItemId={getValue() as number} />
<EditButton hideText size="sm" recordItemId={getValue() as number} />
<DeleteButton
hideText
size="sm"
recordItemId={getValue() as number}
/>
</HStack>
);
},
},
];
export const ProductList = () => {
const {
getHeaderGroups,
getRowModel,
setOptions,
refineCore: {
setCurrent,
pageCount,
current,
tableQuery: { data: tableData },
},
} = useTable<IProduct>({
columns,
refineCoreProps: {
initialSorter: [
{
field: "id",
order: "desc",
},
],
},
});
return (
<List>
<TableContainer whiteSpace="pre-line">
<Table variant="simple">
<Thead>
{getHeaderGroups().map((headerGroup) => (
<Tr key={headerGroup.id}>
{headerGroup.headers.map((header) => (
<Th key={header.id}>
<Text>
{flexRender(
header.column.columnDef.header,
header.getContext(),
)}
</Text>
</Th>
))}
</Tr>
))}
</Thead>
<Tbody>
{getRowModel().rows.map((row) => (
<Tr key={row.id}>
{row.getVisibleCells().map((cell) => (
<Td key={cell.id}>
{flexRender(cell.column.columnDef.cell, cell.getContext())}
</Td>
))}
</Tr>
))}
</Tbody>
</Table>
</TableContainer>
<Pagination
current={current}
pageCount={pageCount}
setCurrent={setCurrent}
/>
</List>
);
};
interface IProduct {
id: string;
name: string;
price: number;
description: string;
}
<Pagination />
component is a custom component that is used to render the pagination controls which uses usePagination
hook from @refinedev/chakra-ui
package. This hook accepts the pagination values from useTable
hook and returns the pagination controls and related props.
Pagination Component
import React from "react";
import { HStack, Button, Box } from "@chakra-ui/react";
import { IconChevronRight, IconChevronLeft } from "@tabler/icons-react";
import { usePagination } from "@refinedev/chakra-ui";
import { IconButton } from "@chakra-ui/react";
type PaginationProps = {
current: number;
pageCount: number;
setCurrent: (page: number) => void;
};
export const Pagination: React.FC<PaginationProps> = ({
current,
pageCount,
setCurrent,
}) => {
const pagination = usePagination({
current,
pageCount,
});
return (
<Box display="flex" justifyContent="flex-end">
<HStack my="3" spacing="1">
{pagination?.prev && (
<IconButton
aria-label="previous page"
onClick={() => setCurrent(current - 1)}
disabled={!pagination?.prev}
variant="outline"
>
<IconChevronLeft size="18" />
</IconButton>
)}
{pagination?.items.map((page) => {
if (typeof page === "string") return <span key={page}>...</span>;
return (
<Button
key={page}
onClick={() => setCurrent(page)}
variant={page === current ? "solid" : "outline"}
>
{page}
</Button>
);
})}
{pagination?.next && (
<IconButton
aria-label="next page"
onClick={() => setCurrent(current + 1)}
variant="outline"
>
<IconChevronRight size="18" />
</IconButton>
)}
</HStack>
</Box>
);
};
Forms
Chakra UI offers form elements yet it does not provide a form management solution. To have a complete solution, Refine recommends using @refinedev/react-hook-form
package which is built on top of Refine's useForm
hook and React Hook Form's useForm
hook.
Refine's documentations and examples of Chakra UI uses @refinedev/react-hook-form
package for form management but you have the option to use any form management solution you want.
import { Create } from "@refinedev/chakra-ui";
import {
FormControl,
FormErrorMessage,
FormLabel,
Input,
Textarea,
} from "@chakra-ui/react";
import { useForm } from "@refinedev/react-hook-form";
export const ProductCreate = () => {
const {
refineCore: { formLoading },
saveButtonProps,
register,
formState: { errors },
} = useForm<IPost>();
return (
<Create isLoading={formLoading} saveButtonProps={saveButtonProps}>
<FormControl mb="3" isInvalid={!!errors?.name}>
<FormLabel>Name</FormLabel>
<Input
id="name"
type="text"
{...register("name", { required: "Name is required" })}
/>
<FormErrorMessage>
{`${errors.name?.message}`}
</FormErrorMessage>
</FormControl>
<FormControl mb="3" isInvalid={!!errors?.material}>
<FormLabel>Material</FormLabel>
<Input
id="material"
type="text"
{...register("material", { required: "Material is required" })}
/>
<FormErrorMessage>
{\`$\{errors.material?.message}\`}
</FormErrorMessage>
</FormControl>
<FormControl mb="3" isInvalid={!!errors?.description}>
<FormLabel>Description</FormLabel>
<Textarea
id="description"
{...register("description", {
required: "Description is required",
})}
/>
<FormErrorMessage>
{\`$\{errors.description?.message}\`}
</FormErrorMessage>
</FormControl>
<FormControl mb="3" isInvalid={!!errors?.price}>
<FormLabel>Price</FormLabel>
<Input
id="price"
type="number"
{...register("price", { required: "Price is required" })}
/>
<FormErrorMessage>
{\`$\{errors.price?.message}\`}
</FormErrorMessage>
</FormControl>
</Create>
);
};
Additional hooks of @refinedev/react-hook-form
such as useStepsForm
and useModalForm
can also be used together with Refine's Chakra UI integration with ease.
Notifications
Chakra UI has its own notification system which works seamlessly with its UI elements. Refine also provides a seamless integration with Chakra UI's notification system and show notifications for related actions and events. This integration is provided by the notificationProvider
hook exported from the @refinedev/chakra-ui
package which can be directly used in the notificationProvider
prop of the <Refine />
component.
import { Refine } from "@refinedev/core";
import { useNotificationProvider } from "@refinedev/chakra-ui";
const App = () => {
return (
<Refine
// ...
notificationProvider={useNotificationProvider}
>
{/* ... */}
</Refine>
);
};
Predefined Components and Views
Layouts, Menus and Breadcrumbs
Refine provides Layout components that can be used to implement a layout for the application. These components are crafted using Chakra UI's components and includes Refine's features and functionalities such as navigation menus, headers, authentication, authorization and more.
- React Router
- Next.js
- Remix
Dependencies: @refinedev/chakra-ui@^2.26.17,@tabler/icons-react@^3.1.0,@refinedev/core@^4.45.1,@refinedev/react-router@latest,@refinedev/simple-rest@^4.5.4,@refinedev/react-table@^5.6.4,@tanstack/react-table@^8.2.6,@refinedev/react-hook-form@^4.8.12,@chakra-ui/react@^2.5.1,react-dom@^18.0.0,react-router@^7.0.2,react-hook-form@^7.43.5
Code Files
Dependencies: @refinedev/chakra-ui@^2.26.17,@tabler/icons-react@^3.1.0,@refinedev/core@^4.45.1,@refinedev/react-router@latest,@refinedev/simple-rest@^4.5.4,@refinedev/react-table@^5.6.4,@tanstack/react-table@^8.2.6,@refinedev/react-hook-form@^4.8.12,@chakra-ui/react@^2.5.1,@refinedev/nextjs-router@latest
Code Files
Dependencies: @refinedev/chakra-ui@^2.26.17,@tabler/icons-react@^3.1.0,@refinedev/core@^4.45.1,@refinedev/react-router@latest,@refinedev/simple-rest@^4.5.4,@refinedev/react-table@^5.6.4,@tanstack/react-table@^8.2.6,@refinedev/react-hook-form@^4.8.12,@chakra-ui/react@^2.5.1,@refinedev/remix-router@latest
Code Files
<ThemedLayoutV2 />
component consists of a header, sider and a content area. The sider have a navigation menu items for the defined resources of Refine, if an authentication provider is present, it will also have a functional logout button. The header contains the app logo and name and also information about the current user if an authentication provider is present.
Additionally, Refine also provides a <Breadcrumb />
component that uses the Chakra UI's component as a base and provide appropriate breadcrumbs for the current route. This component is used in the basic views provided by Refine's Chakra UI package automatically.
Buttons
Refine's Chakra UI integration offers variety of buttons that are built above the <Button />
component of Chakra UI and includes many logical functionalities such as;
- Authorization checks
- Confirmation dialogs
- Loading states
- Invalidation
- Navigation
- Form actions
- Import/Export and more.
You can use buttons such as <EditButton />
or <ListButton />
etc. in your views to provide navigation for the related routes or <DeleteButton />
and <SaveButton />
etc. to perform related actions without having to worry about the authorization checks and other logical functionalities.
An example usage of the <EditButton />
component is as follows:
import React from "react";
import { useTable } from "@refinedev/react-table";
import { ColumnDef, flexRender } from "@tanstack/react-table";
import { GetManyResponse, useMany } from "@refinedev/core";
import { List, EditButton, DateField } from "@refinedev/chakra-ui";
import { Table, Thead, Tbody, Tr, Th, Td, TableContainer, HStack, Text } from "@chakra-ui/react";
const columns = [
{ id: "id", header: "ID", accessorKey: "id" },
{ id: "name", header: "Name", accessorKey: "name", meta: { filterOperator: "contains" } },
{ id: "price", header: "Price", accessorKey: "price" },
{
id: "actions",
header: "Actions",
accessorKey: "id",
cell: function render({ getValue }) {
return (
<EditButton hideText size="sm" recordItemId={getValue() as number} />
);
},
},
];
export const ProductList = () => {
const table = useTable<IProduct>({ columns });
return ( /* ... */ );
};
The list of provided buttons are:
<CreateButton />
<EditButton />
<ListButton />
<ShowButton />
<CloneButton />
<DeleteButton />
<SaveButton />
<RefreshButton />
<ImportButton />
<ExportButton />
Many of these buttons are already used in the views provided by Refine's Chakra UI integration. If you're using the basic view elements provided by Refine, you will have the appropriate buttons placed in your application out of the box.
Views
Views are designed as wrappers around the content of the pages in the application. They are designed to be used within the layouts and provide basic functionalities such as titles based on the resource, breadcrumbs, related actions and authorization checks. Refine's Chakra UI integration uses components such as <Box />
and <Heading />
to provide these views and provides customization options by passing related props to these components.
The list of provided views are:
Dependencies: @refinedev/chakra-ui@^2.26.17,@tabler/icons-react@^3.1.0,@refinedev/core@^4.45.1,@refinedev/react-router@latest,@refinedev/simple-rest@^4.5.4,@refinedev/react-table@^5.6.4,@tanstack/react-table@^8.2.6,@refinedev/react-hook-form@^4.8.12,@chakra-ui/react@^2.5.1,react-dom@^18.0.0,react-router@^7.0.2,react-hook-form@^7.43.5
Code Files
Fields
Refine's Chakra UI also provides field components to render values with appropriate design and format of Chakra UI. These components are built on top of respective Chakra UI components and also provide logic for formatting of the values. While these components might not always be suitable for your use case, they can be combined or extended to provide the desired functionality.
The list of provided field components are:
<BooleanField />
<DateField />
<EmailField />
<FileField />
<MarkdownField />
<NumberField />
<TagField />
<TextField />
<UrlField />
import { useShow } from "@refinedev/core";
import {
Show,
TextField,
NumberField,
MarkdownField,
} from "@refinedev/chakra-ui";
import { Heading } from "@chakra-ui/react";
export const ProductShow = () => {
const { queryResult } = useShow();
const { data, isLoading } = queryResult;
const record = data?.data;
return (
<Show isLoading={isLoading}>
<Heading as="h5" size="sm">
Id
</Heading>
<TextField value={record?.id} />
<Heading as="h5" size="sm" mt={4}>
Name
</Heading>
<TextField value={record?.name} />
<Heading as="h5" size="sm" mt={4}>
Material
</Heading>
<TextField value={record?.material} />
<Heading as="h5" size="sm" mt={4}>
Description
</Heading>
<MarkdownField value={record?.description} />
<Heading as="h5" size="sm" mt={4}>
Price
</Heading>
<NumberField
value={record?.price}
options={{ style: "currency", currency: "USD" }}
/>
</Show>
);
};
Auth Pages
Auth pages are designed to be used as the pages of the authentication flow of the application. They offer an out of the box solution for the login, register, forgot password and reset password pages by leveraging the authentication hooks of Refine. Auth page components are built on top of basic Chakra UI components such as <Input />
and <Card />
etc.
The list of types of auth pages that are available in the UI integrations are:
<AuthPage type="login" />
<AuthPage type="register" />
<AuthPage type="forgot-password" />
<AuthPage type="reset-password" />
An example usage of the <AuthPage />
component is as follows:
Dependencies: @refinedev/chakra-ui@^2.26.17,@tabler/icons-react@^3.1.0,@refinedev/core@^4.45.1,@refinedev/react-router@latest,@refinedev/simple-rest@^4.5.4,@refinedev/react-table@^5.6.4,@tanstack/react-table@^8.2.6,@refinedev/react-hook-form@^4.8.12,@chakra-ui/react@^2.5.1,react-dom@^18.0.0,react-router@^7.0.2,react-hook-form@^7.43.5
Code Files
Error Components
Refine's Chakra UI integration also provides an <ErrorComponent />
component that you can use to render a 404 page in your app. While these components does not offer much functionality, they are provided as an easy way to render an error page with a consistent design language.
An example usage of the <ErrorComponent />
component is as follows:
import { ErrorComponent } from "@refinedev/chakra-ui";
const NotFoundPage = () => {
return <ErrorComponent />;
};
Theming
Since Refine offers application level components such as layout, sidebar and header and page level components for each action, it is important to have it working with the styling of Chakra UI. All components and providers exported from the @refinedev/chakra-ui
package will use the current theme of Chakra UI without any additional configuration.
Additionally, Refine also provides a set of carefully crafted themes for Chakra UI which outputs a nice UI with Refine's components with light and dark theme support. These themes are exported as RefineThemes
object from the @refinedev/chakra-ui
package and can be used in <ChakraProvider />
component of Chakra UI.
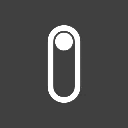
Dependencies: @refinedev/chakra-ui@^2.26.17,@tabler/icons-react@^3.1.0,@refinedev/core@^4.45.1,@refinedev/react-router@latest,@refinedev/simple-rest@^4.5.4,@refinedev/react-table@^5.6.4,@tanstack/react-table@^8.2.6,@refinedev/react-hook-form@^4.8.12,@chakra-ui/react@^2.5.1,react-dom@^18.0.0,react-router@^7.0.2,react-hook-form@^7.43.5
Code Files
To learn more about the theme configuration of Chakra UI, please refer to the official documentation.
Inferencer
You can automatically generate views for your resources using @refinedev/inferencer
. Inferencer exports the ChakraListInferencer
, ChakraShowInferencer
, ChakraEditInferencer
, ChakraCreateInferencer
components and finally the ChakraInferencer
component, which combines all in one place.
To learn more about Inferencer, please refer to the Chakra UI Inferencer docs.