Data Provider
Data provider acts as a data layer for your app, making HTTP requests and encapsulating how the data is retrieved. The methods of these requests are then consumed by Refine via data hooks.
You don’t need to worry about creating data providers from scratch, as Refine offers built-in data provider support for the most popular API providers.
- Data hooks use TanStack Query to manage data fetching, which handles important concerns like caching, invalidation, loading states, etc.
- If you want to create your own data provider, check out the "Creating a data provider from scratch" tutorial
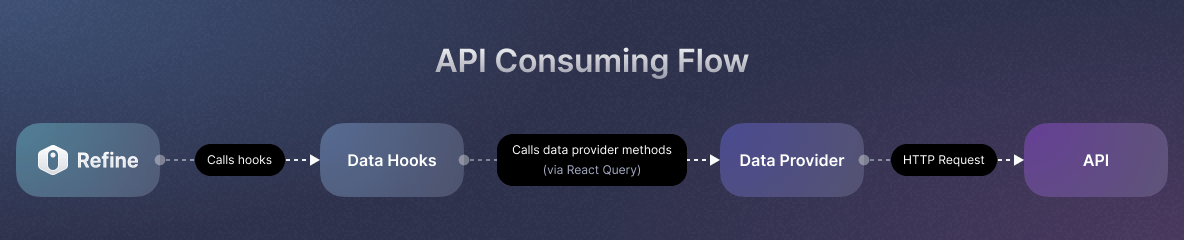
Usage
To activate the data provider in Refine, we have to pass the dataProvider
to the <Refine />
component.
import { Refine } from "@refinedev/core";
import dataProvider from "./dataProvider";
const App = () => (
<Refine
/* ... */
dataProvider={dataProvider}
/>
);
Refer to the Data Provider tutorial for more information and usage examples →
Multiple Data Providers
Refine allows you to use multiple data providers in your app. All you need to do is pass key and value pairs to the dataProvider
prop of the <Refine />
component. In the pair object, the key corresponds to the data provider name, and the value corresponds to the data provider itself.
When defining multiple data providers, default
key is required for defining the default data provider.
Here is an example which uses multiple data providers:
Usage
You can pick data providers in two ways:
You can either use the dataProviderName
prop in data hooks and data-related components/functions:
useTable({
dataProviderName: "example",
});
Or use the meta.dataProviderName
property in your resource config, which will be the default data provider but can be overridden in data hooks and components:
const App = () => (
<Refine
dataProvider={{
default: defaultDataProvider,
example: exampleDataProvider,
}}
resources={[
{
// `default` data provider will be used for this resource
name: "posts",
},
{
name: "products",
// `exampleDataProvider` data provider will be used for this resource
meta: { dataProviderName: "exampleDataProvider" },
},
]}
/>
);
Methods
Data provider's methods are expected to return a promise, meaning that they are async and can be used to create a data provider. Refine consumes these data provider methods using data hooks, which are used for CRUD actions like creating a new record, listing a resource or deleting a record, etc.
import { DataProvider } from "@refinedev/core";
const dataProvider: DataProvider = {
// required methods
getList: ({ resource, pagination, sorters, filters, meta }) => Promise,
create: ({ resource, variables, meta }) => Promise,
update: ({ resource, id, variables, meta }) => Promise,
deleteOne: ({ resource, id, variables, meta }) => Promise,
getOne: ({ resource, id, meta }) => Promise,
getApiUrl: () => "",
// optional methods
getMany: ({ resource, ids, meta }) => Promise,
createMany: ({ resource, variables, meta }) => Promise,
deleteMany: ({ resource, ids, variables, meta }) => Promise,
updateMany: ({ resource, ids, variables, meta }) => Promise,
custom: ({ url, method, filters, sorters, payload, query, headers, meta }) =>
Promise,
};
Refer to the Data Provider tutorial for more information and usage examples→
getList required
The getList
method is used to get a list of resources with sorting, filtering, and pagination features. It takes resource
, sorters
, pagination
, and, filters
as parameters. And it returns both data
and total
fields, regardless of the data provider used.
Refine will consume this method using the useList
or useInfiniteList
data hook.
Retrieving the Total Row Count
- Different data providers have specific ways to determine the total row count, and these are just some examples:
- Simple REST Providers: The
x-total-count
header is commonly used to get the row count. - GraphQL Providers: The total count is often sourced from specific data fields, like
response.data.pageInfo.total
.
- Simple REST Providers: The
This documentation provides only illustrative examples. It's up to the data provider to determine how to best source the total count.
The method signature remains the same, and Refine expects a consistent format:
getList: async ({ resource, pagination, sorters, filters, meta }) => {
const { current, pageSize } = pagination ?? {};
// Adjust request parameters to meet the requirements of your API
const response = await apiClient.get(`/${resource}`, {
params: { _page: current, _limit: pageSize },
});
// The total row count could be sourced differently based on the provider
const total = response.headers["x-total-count"] ?? response.data.length;
return {
data: response.data,
total,
};
};
:::tip
`getList` can also support cursor-based pagination. Refer to [related section in the `useInfiniteList` documentation](/docs/data/hooks/use-infinite-list#how-to-use-cursor-based-pagination) for more information.
:::
**Parameter Types:**
| Name | Type |
| ----------- | ----------------------------- |
| resource | `string` |
| pagination? | [`Pagination`][pagination] |
| sorters? | [`CrudSorting`][crud-sorting] |
| filters? | [`CrudFilters`][crud-filters] |
| meta? | [`MetaDataQuery`][meta-data] |
### create <PropTag required/>
The `create` method creates a new record with the `resource` and `variables` parameters.
Refine will consume this method using the [`useCreate`][use-create] data hook.
```ts
create: async ({ resource, variables, meta }) => {
// You can handle the request according to your API requirements.
return {
data,
};
};
Parameter Types
Name | Type | Default |
---|---|---|
resource | string | |
variables | TVariables | {} |
meta? | MetaDataQuery |
TVariables
is a user defined type which can be passed touseCreate
to typevariables
.
update required
The update
method updates the record with the resource
, id
, and, variables
parameters.
Refine will consume this method using the useUpdate
data hook.
update: async ({ resource, id, variables, meta }) => {
// You can handle the request according to your API requirements.
return {
data,
};
};
Parameter Types:
Name | Type | Default |
---|---|---|
resource | string | |
id | BaseKey | |
variables | TVariables | {} |
meta? | MetaDataQuery |
TVariables
is a user defined type which can be passed touseUpdate
to typevariables
.
deleteOne required
The deleteOne
method delete the record with the resource
and id
parameters.
Refine will consume this method using the useDelete
data hook.
deleteOne: async ({ resource, id, variables, meta }) => {
// You can handle the request according to your API requirements.
return {
data,
};
};
Parameter Types:
Name | Type | Default |
---|---|---|
resource | string | |
id | BaseKey | |
variables | TVariables[] | {} |
meta? | MetaDataQuery |
TVariables
is a user defined type which can be passed touseDelete
to typevariables
.
getOne required
The getOne
method gets the record with the resource
and id
parameters.
Refine will consume this method using the useOne
data hook.
getOne: async ({ resource, id, meta }) => {
// You can handle the request according to your API requirements.
return {
data,
};
};
Parameter Types:
Name | Type | Default |
---|---|---|
resource | string | |
id | BaseKey | |
meta? | MetaDataQuery |
getApiUrl required
The getApiUrl
method returns the apiUrl
value.
Refine will consume this method using the useApiUrl
data hook.
import { DataProvider } from "@refinedev/core";
export const dataProvider = (apiUrl: string): DataProvider => ({
getApiUrl: () => apiUrl,
// ...
});
custom
An optional method named custom
can be added to handle requests with custom parameters like URL, CRUD methods and configurations.
It's useful if you have non-standard REST API endpoints or want to make a connection with external resources.
Refine will consume this method using the useCustom
data hook.
custom: async ({
url,
method,
filters,
sorters,
payload,
query,
headers,
meta,
}) => {
// You can handle the request according to your API requirements.
return {
data,
};
};
Parameter Types
Name | Type |
---|---|
url | string |
method | get , delete , head , options , post , put , patch |
sorters? | CrudSorting |
filters? | CrudFilters |
payload? | {} |
query? | {} |
headers? | {} |
meta? | MetaDataQuery |
Bulk Actions
Bulk actions are actions that can be performed on multiple items at once to improve speed and efficiency. They are commonly used in admin panels. They can be used for data import
and export
, and are also atomic, meaning that they are treated as a single unit.
If your API supports bulk actions, you can implement them in your data provider.
getMany
The getMany
method gets the records with the resource
and ids
parameters. This method is optional, and Refine will use the getOne
method to handle multiple requests if you don't implement it.
Refine will consume this getMany
method using the useMany
data hook.
getMany: async ({ resource, ids, meta }) => {
// You can handle the request according to your API requirements.
return {
data,
};
};
Parameter Types:
Name | Type | Default |
---|---|---|
resource | string | |
ids | [BaseKey] | |
meta? | MetaDataQuery |
createMany
This method allows us to create multiple items in a resource. This method is optional, and Refine will use the create
method to handle multiple requests if you don't implement it.
Refine will consume this createMany
method using the useCreateMany
data hook.
createMany: async ({ resource, variables, meta }) => {
// You can handle the request according to your API requirements.
return {
data,
};
};
Parameter Types:
Name | Type | Default |
---|---|---|
resource | string | |
variables | TVariables[] | {} |
meta? | MetaDataQuery |
TVariables
is a user defined type which can be passed touseCreateMany
to typevariables
.
deleteMany
This method allows us to delete multiple items in a resource. This method is optional, and Refine will use the deleteOne
method to handle multiple requests if you don't implement it.
Refine will consume this deleteMany
method using the useDeleteMany
data hook.
deleteMany: async ({ resource, ids, variables, meta }) => {
// You can handle the request according to your API requirements.
return {
data,
};
};
Name | Type | Default |
---|---|---|
resource | string | |
ids | [BaseKey] | |
variables | TVariables[] | {} |
meta? | MetaDataQuery |
TVariables
is a user defined type which can be passed touseDeleteMany
to typevariables
.
updateMany
This method allows us to update multiple items in a resource. This method is optional, and Refine will use the update
method to handle multiple requests if you don't implement it.
Refine will consume this updateMany
method using the useUpdateMany
data hook.
updateMany: async ({ resource, ids, variables, meta }) => {
// You can handle the request according to your API requirements.
return {
data,
};
};
Name | Type | Default |
---|---|---|
resource | string | |
ids | [BaseKey] | |
variables | TVariables[] | {} |
meta? | MetaDataQuery |
TVariables
is a user defined type which can be passed touseUpdateMany
to typevariables
.
Error Format
Refine expects errors to be extended from HttpError
.
Here is a basic example of how to implement error handling in your data provider.
import { DataProvider, HttpError } from "@refinedev/core";
export const dataProvider = (apiUrl: string): DataProvider => ({
getOne: async ({ resource, id }) => {
try {
const response = await fetch(`https://api.example.com/${resource}/${id}`);
if (!response.ok) {
const error: HttpError = {
message: response.statusText,
statusCode: response.status,
};
return Promise.reject(error);
}
return {
data: response.data,
};
} catch (error) {
const error: HttpError = {
message: error?.message || "Something went wrong",
statusCode: error?.status || 500,
};
return Promise.reject(error);
}
},
// ...
});
Also, the Axios interceptor can be used to transform the error from the response before Axios returns the response to your code.
import axios from "axios";
import { DataProvider, HttpError } from "@refinedev/core";
import { stringify } from "query-string";
// Error handling with axios interceptors
const axiosInstance = axios.create();
axiosInstance.interceptors.response.use(
(response) => {
return response;
},
(error) => {
const customError: HttpError = {
...error,
message: error.response?.data?.message,
statusCode: error.response?.status,
};
return Promise.reject(customError);
},
);
export const dataProvider = (apiUrl: string): DataProvider => ({
// Methods
});
meta Usage
When using APIs, you may wish to include custom parameters, such as a custom header. To accomplish this, you can utilize the meta
field, which allows the sent parameter to be easily accessed by the data provider.
Here is an example of how to send a custom header parameter to the getOne
method using meta
:
We first need to send a custom header parameter to the getOne
method using meta
.
import { useOne } from "@refinedev/core";
useOne({
resource: "post",
id: "1",
meta: {
headers: {
"x-custom-header": "hello world",
},
},
});
Then we can get the meta
parameter from the data provider:
import { DataProvider } from "@refinedev/core";
export const dataProvider = (apiUrl: string): DataProvider => ({
...
getOne: async ({ resource, id, variables, meta }) => {
const { headers } = meta;
const url = `${apiUrl}/${resource}/${id}`;
httpClient.defaults.headers = {
...headers,
};
const { data } = await httpClient.get(url, variables);
return {
data,
};
},
});
Supported Data Providers
Refine supports many data providers. To include them in your project, you can use npm install [packageName]
or you can select the preferred data provider with the npm create refine-app@latest projectName
during the project creation phase with CLI. This will allow you to easily use these data providers in your project.
Community ❤️
- Firebase by rturan29
- Directus by tspvivek
- Elide by chirdeeptomar
- Elide GraphQL by chirdeeptomar
- useGenerated by usegen
- Hygraph by acomagu
- Sanity by hirenf14
- SQLite by mateusabelli
- JSON:API by mahirmahdi
- PocketBase by kruschid
If you have created a custom data provider and would like to share it with the community, please don't hesitate to get in touch with us. We would be happy to include it on this page for others to use.
Supported Hooks
Refine will consume:
getList
method using theuseList
oruseInfiniteList
data hook.create
method using theuseCreate
data hook.update
method using theuseUpdate
data hook.deleteOne
method using theuseDeleteOne
data hook.getOne
method using theuseOne
data hook.getApiUrl
method using theuseApiUrl
data hook.custom
method using theuseCustom
data hook.getMany
method using theuseMany
data hook.createMany
method using theuseCreateMany
data hook.deleteMany
method using theuseDeleteMany
data hook.updateMany
method using theuseUpdateMany
data hook.
FAQ
How can I create a custom data provider?
How can I customize existing data providers?
How I can override a specific method of Data Providers?
In some cases, you may need to override the method of Refine data providers. The simplest way to do this is to use the Spread syntax
For example, Let's override the update
function of the @refinedev/simple-rest
. @refinedev/simple-rest
uses the PATCH
HTTP method for update
, let's change it to PUT
without forking the whole data provider.
import dataProvider from "@refinedev/simple-rest";
const simpleRestProvider = dataProvider("API_URL");
const myDataProvider = {
...simpleRestProvider,
update: async ({ resource, id, variables }) => {
const url = `${apiUrl}/${resource}/${id}`;
const { data } = await httpClient.put(url, variables);
return {
data,
};
},
};
<Refine dataProvider={myDataProvider}>{/* ... */}</Refine>;
- Usage
- Multiple Data Providers
- Usage
- Methods
- getList
- Retrieving the Total Row Count
- update
- deleteOne
- getOne
- getApiUrl
- custom
- Bulk Actions
- getMany
- createMany
- deleteMany
- updateMany
- Error Format
- meta Usage
- Supported Data Providers
- Supported Hooks
- FAQ
- How can I create a custom data provider?
- How can I customize existing data providers?
- How I can override a specific method of Data Providers?